Why Drupal Developers Should Start Using PHP Attributes Over Annotations
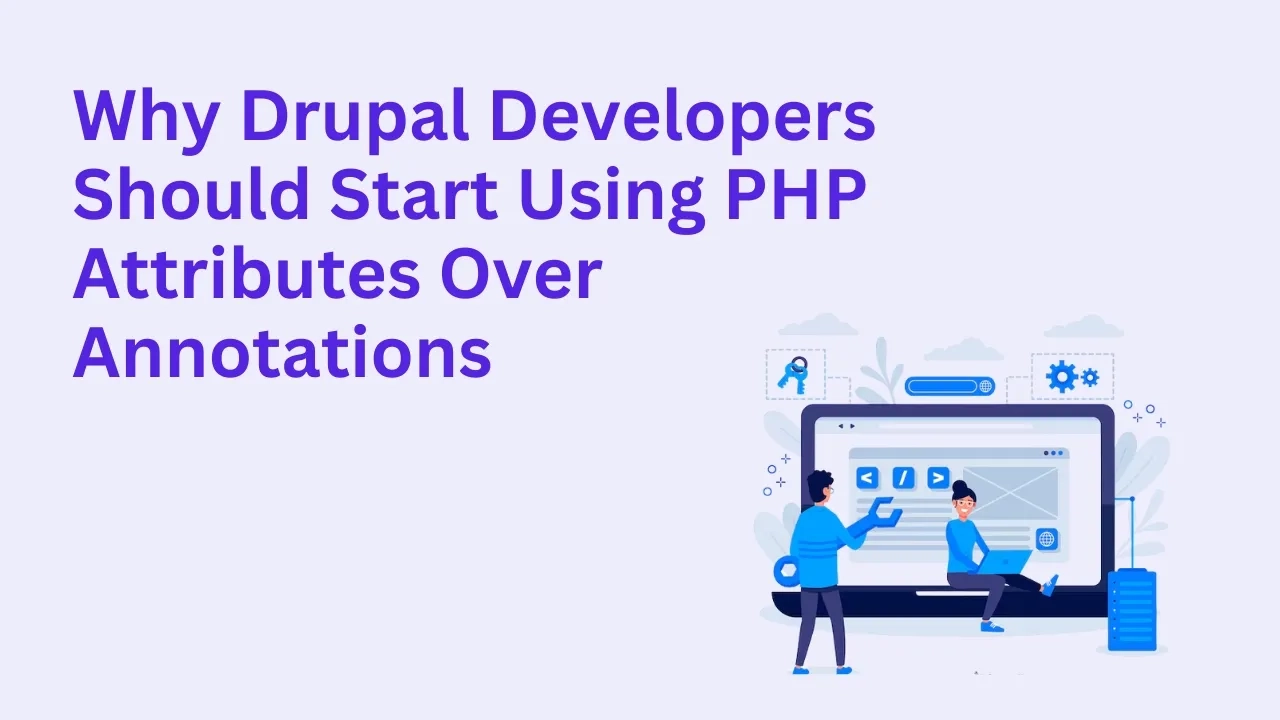
With the release of PHP 8, attributes have emerged as a modern and structured alternative to annotations. For Drupal developers, transitioning to attributes can bring significant benefits, improving code readability, maintainability, and performance.
What Are PHP Attributes?
PHP attributes are a built-in way to add metadata to classes, methods, properties, and parameters. Unlike annotations, which rely on docblocks and require parsing, attributes are part of PHP’s core syntax and can be processed natively.
Example of PHP Attributes
use Drupal\Core\DependencyInjection\ContainerInjectionInterface;
use Symfony\Component\DependencyInjection\ContainerInterface;
use Attribute;
#[Attribute]
class CustomAttribute {
public function __construct(
public string $message
) {}
}
class ExampleService implements ContainerInjectionInterface {
#[CustomAttribute("This is a custom attribute")]
public function exampleFunction() {
// Function logic here
}
public static function create(ContainerInterface $container) {
return new static();
}
}
Why Switch to PHP Attributes?
1. Better Performance
PHP attributes eliminate the need to parse annotations from docblocks, reducing overhead and improving runtime efficiency.
2. Improved Readability & Maintainability
Attributes provide a structured way to define metadata directly in code, making it more readable and easier to maintain.
3. Native PHP Support
Unlike annotations, attributes are built into PHP itself, ensuring better support and fewer dependencies on third-party libraries.
4. Stronger Type Safety
Attributes utilize PHP classes and constructors, allowing for type hints and better validation at compile time.
How to Migrate from Annotations to Attributes
Example of Doctrine Annotations:
/**
* @Route("/custom-route", name="custom_route")
*/
public function customMethod() {
// Method logic
}
Equivalent PHP Attribute:
use Symfony\Component\Routing\Annotation\Route;
#[Route("/custom-route", name: "custom_route")]
public function customMethod() {
// Method logic
}
Considerations Before Migrating
- Drupal Compatibility: Ensure that all modules and dependencies support PHP attributes.
- Backwards Compatibility: If maintaining older projects, annotations might still be required for compatibility with legacy systems.
- Testing & Debugging: Thoroughly test code after migration to ensure smooth functionality.
Conclusion
PHP attributes offer a modern, efficient, and structured approach to metadata in Drupal development. By adopting attributes over annotations, Drupal developers can benefit from improved performance, readability, and maintainability, making their codebase future-proof and easier to manage.