What Are Custom Services in Drupal & How to Override Them?
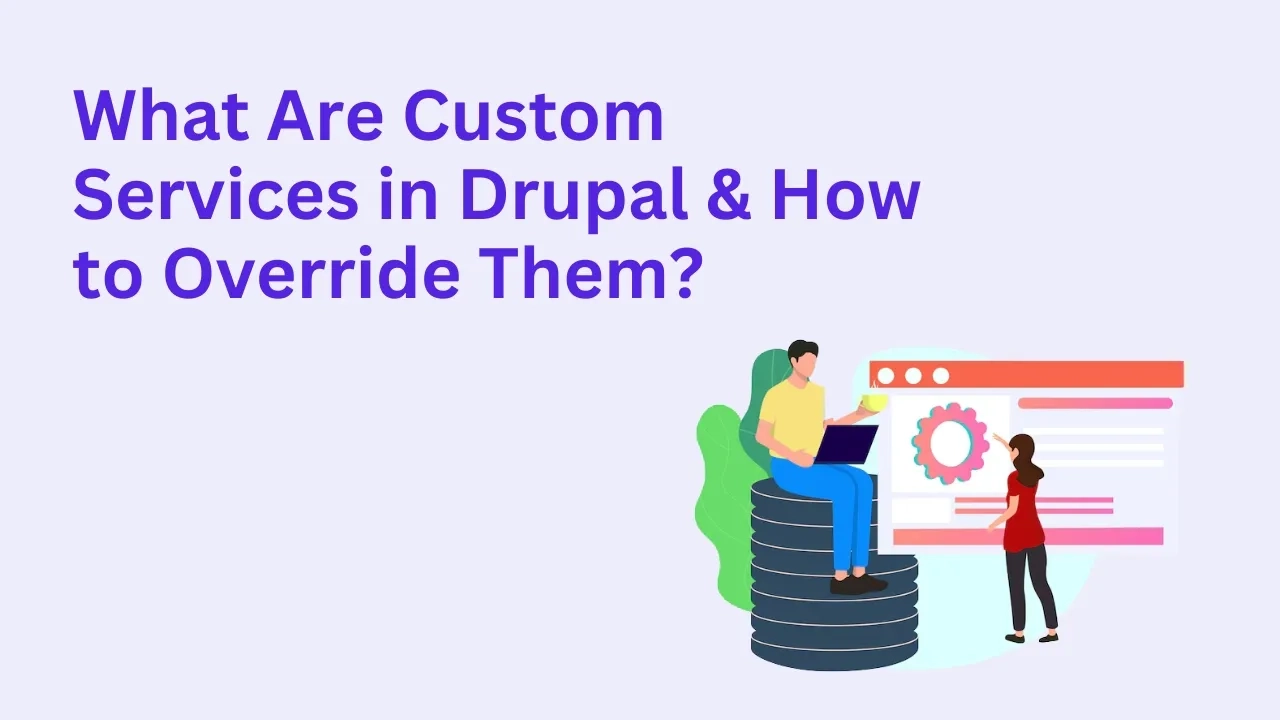
Drupal's service container provides a powerful way to manage reusable functionality throughout a website. Understanding custom services and how to override them is essential for developers looking to enhance Drupal's capabilities.
What Are Custom Services in Drupal?
In Drupal, services are reusable pieces of functionality defined using the Symfony service container. These services allow developers to separate logic from controllers, hooks, and plugins, making the code more maintainable and modular.
Why Use Custom Services?
- Code Reusability: Services encapsulate logic that can be used across different parts of a Drupal site.
- Improved Maintainability: Code is cleaner and easier to manage.
- Dependency Injection: Services allow for better control over dependencies rather than using static methods or global variables.
Defining a Custom Service
To create a custom service in Drupal, follow these steps:
Define the Service in a
.services.yml
File
Each module can have its own service definition file, typically found at:my_module.services.yml
Example definition:
services: my_module.custom_service: class: Drupal\my_module\Services\CustomService arguments: ['@logger.factory']
Create the Service Class
The service class is where the logic is defined. Example:namespace Drupal\my_module\Services; use Psr\Log\LoggerInterface; class CustomService { protected $logger; public function __construct(LoggerInterface $logger) { $this->logger = $logger; } public function logMessage($message) { $this->logger->info($message); } }
Use the Custom Service
Inject the service into a controller, block, or another service using dependency injection:use Drupal\my_module\Services\CustomService; class MyController { protected $customService; public function __construct(CustomService $customService) { $this->customService = $customService; } public function someFunction() { $this->customService->logMessage('Custom service used!'); } }
How to Override a Service in Drupal
Sometimes, you may need to override an existing service to modify its behavior. Drupal allows you to do this by redefining the service in your module’s .services.yml
file.
Steps to Override a Service
Identify the Original Service
Find the service you want to override by checking Drupal’s service list:drush debug:container | grep service_name
Redefine the Service in Your Module's
services.yml
Fileservices: existing.service_name: class: Drupal\my_module\Services\CustomOverrideService arguments: ['@another_service']
Create the Custom Override Class
namespace Drupal\my_module\Services; use Original\Service\Class; class CustomOverrideService extends Class { public function modifiedFunction() { // Custom logic overriding original functionality } }
Best Practices for Overriding Services
- Use Service Decoration: Instead of completely overriding a service, consider decorating it to extend functionality while preserving original behavior.
- Keep Dependency Injection in Mind: Ensure your overridden service maintains proper dependencies.
- Clear Cache: Run
drush cache:rebuild
after making changes to apply updates.
Conclusion
Drupal’s service architecture allows developers to create reusable and maintainable code. Custom services help structure functionality efficiently, while overriding services enables customization without altering the core code. By following best practices, you can extend Drupal’s capabilities while keeping your projects flexible and future-proof.