PHP Design Patterns in Drupal: The Only Guide You Need!
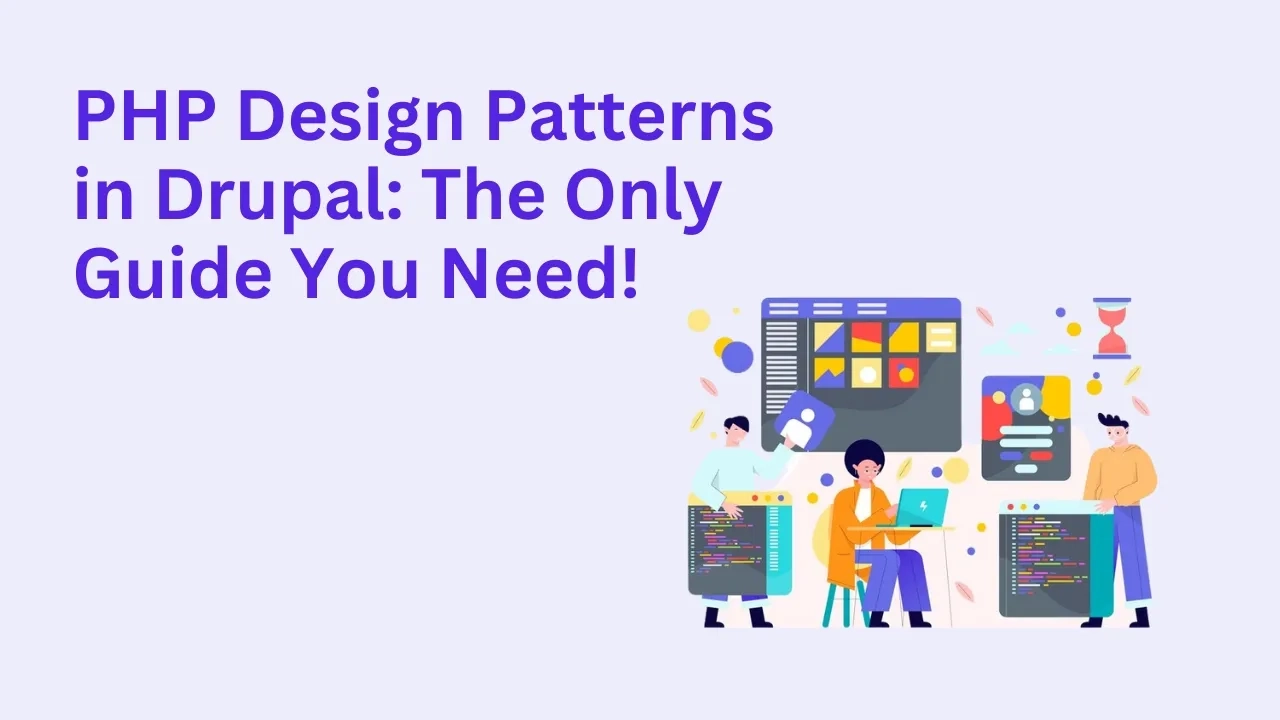
PHP design patterns are essential tools for building scalable and maintainable applications. In Drupal, leveraging these patterns effectively ensures better performance and extensibility. This guide dives deep into how PHP design patterns are used in Drupal, providing practical insights and examples.
What Are PHP Design Patterns?
Design patterns are reusable solutions to common software design problems. In PHP, these patterns provide developers with standardized methods for structuring code, ensuring consistency, and improving collaboration. They are particularly valuable in complex systems like Drupal, where modularity and flexibility are crucial.
Key Benefits of Using PHP Design Patterns in Drupal:
- Improved Code Readability: Easier for teams to understand and collaborate.
- Scalability: Patterns ensure your Drupal application can grow with your business needs.
- Reusability: Common solutions can be implemented across different projects.
- Maintainability: Organized code reduces the risk of bugs and simplifies updates.
Why Are Design Patterns Important in Drupal?
Drupal, being a robust CMS, relies heavily on design principles to provide flexibility and extensibility. Design patterns play a critical role in:
- Module Development: Simplifying the process of creating reusable modules.
- Theming: Ensuring a consistent approach to building themes.
- Performance Optimization: Structuring code for faster execution.
- Custom Functionality: Providing a framework for building tailored solutions.
Also read: How to Setup a Drupal Site on Pantheon from Scratch
Common PHP Design Patterns Used in Drupal
Drupal incorporates several design patterns to solve specific challenges. Below, we’ll explore some of the most commonly used patterns in Drupal development.
1. Singleton Pattern
The Singleton pattern ensures that a class has only one instance, providing a global point of access to it. In Drupal, this pattern is often used for managing services like database connections.
Example:
class DatabaseConnection {
private static $instance;
private function __construct() {}
public static function getInstance() {
if (self::$instance === null) {
self::$instance = new self();
}
return self::$instance;
}
}
In Drupal, services like configuration management use a similar approach to maintain consistency.
2. Factory Pattern
The Factory pattern provides a way to create objects without specifying their concrete classes. This is useful for creating flexible and extensible modules in Drupal.
Use Case in Drupal:
The service container in Drupal implements the Factory pattern to manage dependency injection, ensuring modules can dynamically access services.
3. Observer Pattern
The Observer pattern allows objects to be notified when changes occur in another object. In Drupal, this is commonly used in event handling and hook systems.
Example:
- Hooks: Drupal’s hooks system notifies modules of specific events, such as hook_node_insert() when a new node is created.
4. Decorator Pattern
The Decorator pattern dynamically adds behavior to objects without modifying their structure. In Drupal, this is evident in the use of render arrays and preprocess functions.
5. Dependency Injection Pattern
Dependency Injection allows objects to depend on abstractions rather than concrete implementations. Drupal’s service container uses this pattern extensively.
Example:
namespace Drupal\example_module;
use Symfony\Component\DependencyInjection\ContainerInterface;
class ExampleService {
protected $logger;
public function __construct($logger) {
$this->logger = $logger;
}
public static function create(ContainerInterface $container) {
return new static(
$container->get('logger.factory')->get('example_module')
);
}
}
How to Implement PHP Design Patterns in Drupal Development
Step 1: Understand Your Requirements
Before implementing a pattern, identify the problem you are trying to solve and assess whether the pattern fits.
Also read: Starterkit Theme in Drupal 10
Step 2: Use Drupal’s Built-In Features
Drupal provides many tools and frameworks that already implement design patterns. Leverage these to save time and ensure best practices.
Step 3: Follow Coding Standards
Adhere to Drupal’s coding standards to ensure compatibility and maintainability.
Step 4: Test Extensively
Test your implementation to identify any potential issues early.
Real-World Examples of Design Patterns in Drupal
- Configuration Management:
- Implements Singleton and Dependency Injection patterns.
- Entity API:
- Leverages Factory and Repository patterns for managing content entities.
- Views Module:
- Uses the Observer pattern for reacting to data changes.
Best Practices for Using Design Patterns in Drupal
- Choose Patterns Wisely: Not every problem requires a design pattern.
- Keep It Simple: Avoid over-engineering your solution.
- Leverage the Community: Drupal’s community provides extensive documentation and examples.
- Stay Updated: Regularly update your knowledge to keep pace with new developments.
Conclusion
Understanding and implementing PHP design patterns in Drupal is crucial for building robust, scalable, and maintainable websites. By mastering these patterns, you can enhance your development workflow and deliver high-quality projects. Start leveraging design patterns in your Drupal development today and experience the difference!